Print of www.smithonline.id.au on Tuesday 01Jul2025 Page : Wireless Control
PowerTran Remote Control Mains Operated Switch
For my Arduino home controller project, I purchased a Powertran Remote Control Mains Operated Switch from Altronics - 1 remote control and 4 mains switches. Several people have described this or similar projects to interface to Arduino systems using relays across the remote buttons. But since the device uses standard 433Mhz wireless I wanted to see if I could dispense with the remote altogether.
I am a bit concerned that there is no "system or site unique" identifier
capability provided by the Powertrans remotes. So if one of my
neighbours buys the same remotes then I could have a problem with my
remotes being overriden. I'd prefer to be able to setup some sort of
code that defines my group of remotes.
Looking at the remote, a LX2262A-4 chip is used to create a bit stream to the 433Mhz modulator. Using a CRO to examine the logic waveform to the modulator shows that the bit stream is an 8 bit address followed by 8 bit blank followed on an on or off command as follows. A "0" bit is 250us on followed by 750us off, while an "1" bit is 750us on followed by 250us off. The signal levels are 12V to the modulator through a 47K resistor.
The following pictures show the results of my investigation. There's not too much English data available for the LX2262 chip.
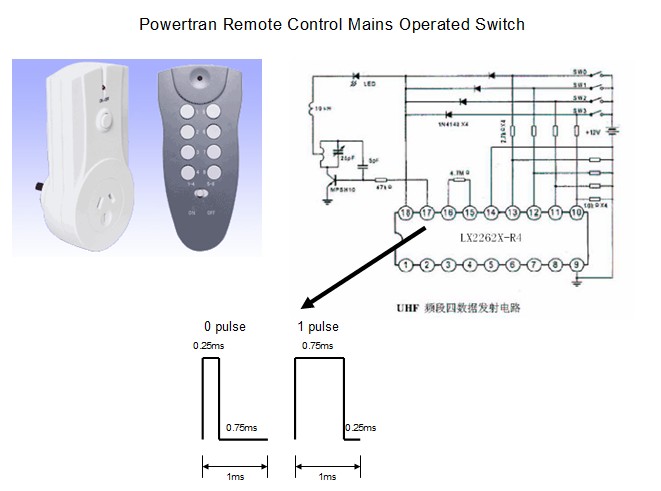
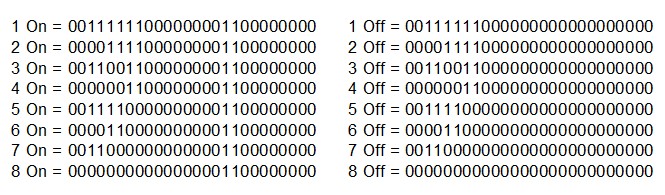
My intention was to see if I could get my Arduino system to generate the waverform directly into a 433Mhz modulator / transmitter also purchased from Altronics.
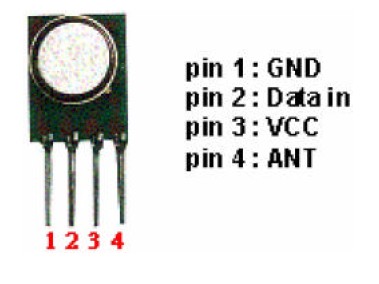
Well the final result works well and have enough range to cover the whole house. Here's the final circuit and code I used.
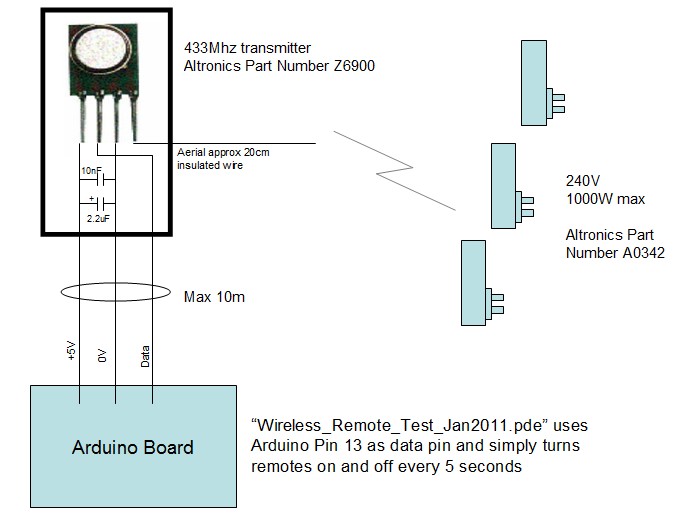
Here's a test program. The real important part is the routine "remoteControl" which takes parameters on channel number (1 to 8) and command (0=off, 1=on).
/*
Wireless_Remote_Test_Jan2011
This program contains the routine remoteControl which sends a
series of bits to the 433Mhz modulator to turn remotes on or off.
The output used for the data output is defined as radioPin
Ideally, the command to turn on or off remotes should be sent when
required, and also every minute or so afterwards. This is because
the remote may not get the instruction the first time, so
continuous repitition is a good idea. However the signal should
not be sent continuously because other 433Mhz appliances around
the house (car remote, garage door, weather station) may stop
working.
Tuning: Sometimes it may be desirable to tune some parameters to
improve performance and range. The parameters shortTime and longTime
in routine remoteControl adjust the pulse timing. If comms are a
bit sketchy, try sending each packet more times (it send it four
times now. The delay after sending each packet can also be adjusted.
*/
#define radioPin 13 // IO number for radio output
void setup() {
pinMode(radioPin, OUTPUT); // set data to transmitter pin to output
}
void loop() {
remoteControl(1,0); // Turn Remote 1 off
remoteControl(2,0); // Turn Remote 2 off
remoteControl(3,0); // Turn Remote 3 off
remoteControl(4,0); // Turn Remote 4 off
remoteControl(5,0); // Turn Remote 5 off
remoteControl(6,0); // Turn Remote 6 off
remoteControl(7,0); // Turn Remote 7 off
remoteControl(8,0); // Turn Remote 8 off
delay(5000); // Delay 5 seconds
remoteControl(1,1); // Turn Remote 1 on
remoteControl(2,1); // Turn Remote 2 on
remoteControl(3,1); // Turn Remote 3 on
remoteControl(4,1); // Turn Remote 4 on
remoteControl(5,1); // Turn Remote 5 on
remoteControl(6,1); // Turn Remote 6 on
remoteControl(7,1); // Turn Remote 7 on
remoteControl(8,1); // Turn Remote 8 on
delay(5000); // Delay 5 seconds
}
void remoteControl(int channelNum, boolean remoteState) {
// Turns on or off remote channel
//
// channelNum : 1 to 8 remote channel number (set on wireless plugpack
// remoteState : 0 = Off, 1 = On
//
// Sends same packet signal 4 times
// 13 Bit pattern in remoteOn and remoteOff arrays
int longTime = 740; // microseconds
int shortTime = 240; // microseconds
unsigned int remoteOn[8] = {0x010E, // 0000 0001 0000 1110
0x010C, // 0000 0001 0000 1100
0x010A, // 0000 0001 0000 1010
0x0108, // 0000 0001 0000 1000
0x0106, // 0000 0001 0000 0110
0x0104, // 0000 0001 0000 0100
0x0102, // 0000 0001 0000 0010
0x0100}; // 0000 0001 0000 0000
unsigned int remoteOff[8] = {0x000E, // 0000 0000 0000 1110
0x000C, // 0000 0000 0000 1100
0x000A, // 0000 0000 0000 1010
0x0008, // 0000 0000 0000 1000
0x0006, // 0000 0000 0000 0110
0x0004, // 0000 0000 0000 0100
0x0002, // 0000 0000 0000 0010
0x0000}; // 0000 0000 0000 0000
// "0" is 240us on, 740us off, 240us on, 740us off
// "1" is 740us on, 240us off, 740us on, 240us off
// Send order is bit0, bit1, bit2 ... bit 12
// Now send packet four times
for (int j=0; j<=4; j++) {
if (remoteState) { // Turn on
for (int k=0; k<=12; k++) { // Send 13 bits in total
if (!bitRead(remoteOn[channelNum-1],k)) {
digitalWrite(radioPin, HIGH); // "0"
delayMicroseconds(shortTime);
digitalWrite(radioPin, LOW);
delayMicroseconds(longTime);
digitalWrite(radioPin, HIGH);
delayMicroseconds(shortTime);
digitalWrite(radioPin, LOW);
delayMicroseconds(longTime);
} else {
digitalWrite(radioPin, HIGH); // "1"
delayMicroseconds(longTime);
digitalWrite(radioPin, LOW);
delayMicroseconds(shortTime);
digitalWrite(radioPin, HIGH);
delayMicroseconds(longTime);
digitalWrite(radioPin, LOW);
delayMicroseconds(shortTime);
}
}
} else { // Turn off
for (int k=0; k<=12; k++) { // Send 13 bits in total
if (!bitRead(remoteOff[channelNum-1],k)) {
digitalWrite(radioPin, HIGH); // "0"
delayMicroseconds(shortTime);
digitalWrite(radioPin, LOW);
delayMicroseconds(longTime);
digitalWrite(radioPin, HIGH);
delayMicroseconds(shortTime);
digitalWrite(radioPin, LOW);
delayMicroseconds(longTime);
} else {
digitalWrite(radioPin, HIGH); // "1"
delayMicroseconds(longTime);
digitalWrite(radioPin, LOW);
delayMicroseconds(shortTime);
digitalWrite(radioPin, HIGH);
delayMicroseconds(longTime);
digitalWrite(radioPin, LOW);
delayMicroseconds(shortTime);
}
}
}
delay(5); // Short delay between packets
}
delay(10); // short delay after sending
}
The arduino project file (.pde) for above can be downloaded here.
Note it's important not to transmit all the time (as I found out with playing with a previous PICAXE implementation). Lots of things work on 433Mhz including car remotes, garage remotes, weather stations, etc. Each will have it's own transmission pattern/code to avoid interference - but you still need to allow enough air-space/time for all these devices to share. (When I tried my PICAXE based wireless system transmitting all the time, my car refused to open and the neighbours weather station died - transmitting every 15 seconds fixed that ok.) Also that's the rational for transmitting a command several times. Just sending once may not work because the transmission may get garbled by other devices using the airspace at the same time.
Print of www.smithonline.id.au on Tuesday 01Jul2025